Updated to Ghost 2.1.0
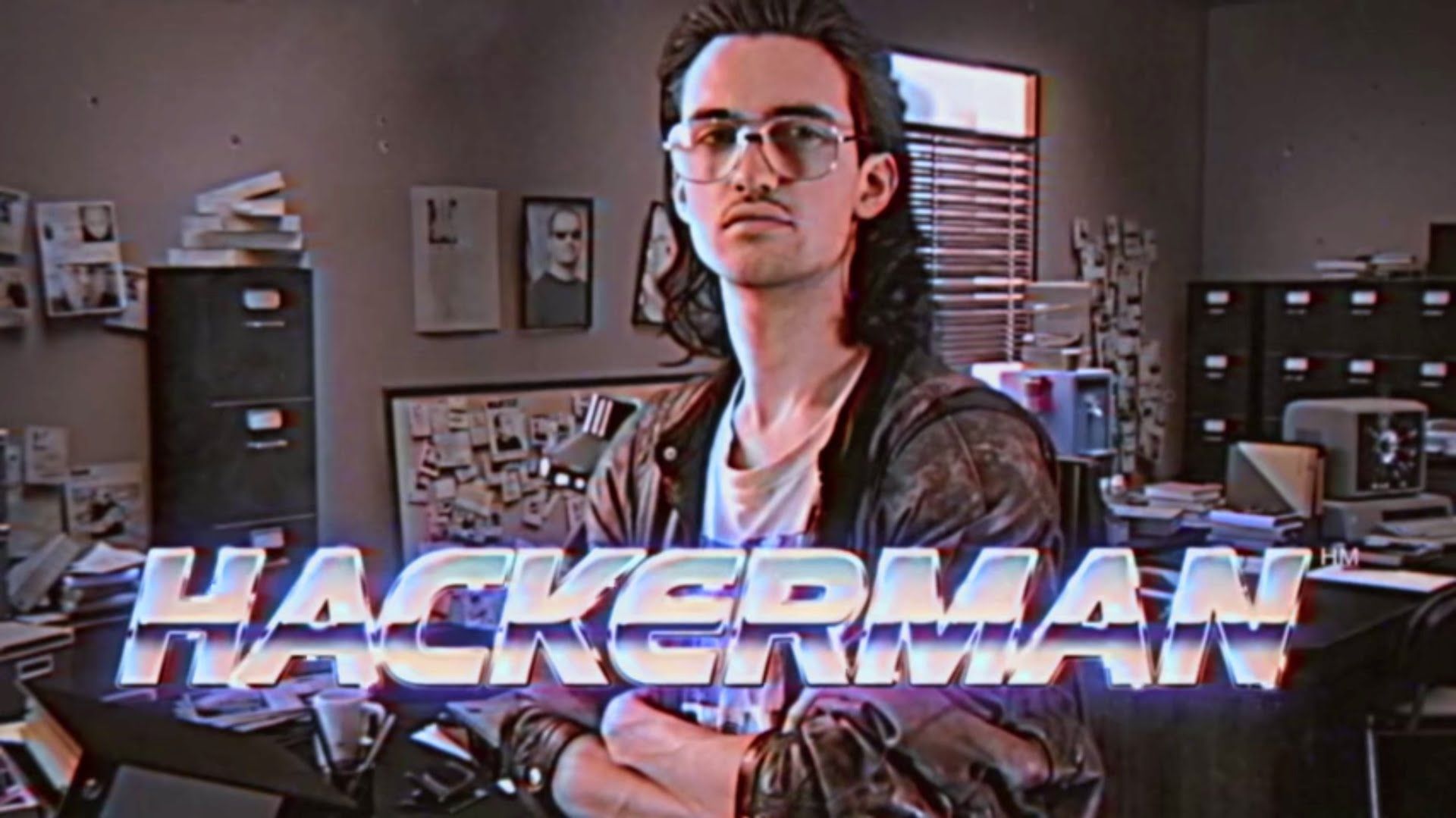
Well, I haven't been writing much lately. Which is understandable, given how much I suck at being consistent with site updates.
But I found a bit of time to update to the latest version of Ghost. It was a bit of a pain, because so much time it passed since last time that the new version didn't even accept the import data from my old one, so I had to create a script to translate the json from my version (I was in version code "004" according to the exported json file) to the new one.
I haven't cleaned it up or anything but it might help someone, so I'm gonna drop my bit of code here:
const loadJsonFile = require('load-json-file');
const converter = require('@tryghost/html-to-mobiledoc');
const fs = require('fs');
function migrateUser(user) {
return {
id: user.id,
name: user.name,
slug: user.slug,
email: user.email,
profile_image: user.image,
cover_image: user.cover,
bio: user.bio,
website: user.website,
location: user.location,
accessibility: user.accessibility,
status: user.status,
language: user.language,
meta_title: user.meta_title,
meta_description: user.meta_description,
last_login: user.last_login,
created_at: user.created_at,
created_by: user.created_by,
updated_at: user.updated_at,
updated_by: user.updated_by
};
}
function migratePost(post) {
const mobiledoc = JSON.stringify(converter.toMobiledoc(post.html));
return {
id: post.id,
title: post.title,
slug: post.slug,
mobiledoc: mobiledoc,
html: post.html,
feature_image: post.image,
featured: post.featured,
page: post.page,
status: post.status,
language: post.language,
meta_title: post.meta_title,
meta_description: post.meta_description,
author_id: post.author_id,
created_at: post.created_at,
created_by: post.created_by,
updated_at: post.updated_at,
updated_by: post.updated_by,
published_at: post.published_at,
published_by: post.published_by
};
}
// change your file name here
loadJsonFile('nacho-lopez.ghost.2018-10-24.json').then(json => {
const migration = {};
// meta
migration.meta = {
exported_on: 1540415441619,
version: "2.1.0"
};
const newData = {};
migration.data = newData;
const originalData = json.db[0].data;
// users
const newUsers = [];
newData.users = newUsers;
migration.data = newData;
originalData.users.map((user) => {
newUsers.push(migrateUser(user));
});
// tags
const newTags = [];
newData.tags = newTags;
originalData.tags.map((tag) => {
newTags.push({
id: tag.id,
name: tag.name,
slug: tag.slug,
description: tag.description
});
});
// posts tags
const newPostsTags = [];
newData.posts_tags = newPostsTags;
originalData.posts_tags.map((postTag) => {
newPostsTags.push({
tag_id: postTag.tag_id,
post_id: postTag.post_id
});
});
// posts
const newPosts = [];
newData.posts = newPosts;
originalData.posts.map((post) => {
newPosts.push(migratePost(post));
});
fs.writeFile("migrated_data.json", JSON.stringify(migration), (err) =>{
if (err) {
console.log("There was an error: ", err);
} else {
console.log("Migration written to migrated_data.json");
}
});
});
And the package.json, for your sweet dependencies:
{
"name": "migrate-ghost",
"version": "1.0.0",
"description": "Migrating between ghost pre 1.0 and 2.0",
"main": "index.js",
"author": "Nacho Lopez",
"license": "MIT",
"dependencies": {
"@tryghost/html-to-mobiledoc": "^0.1.2",
"load-json-file": "^5.1.0"
}
}
I am sure there are multiple old articles with broken syntax highlighting, my bad. I fixed it manually in the ones with more activity, but the rest...
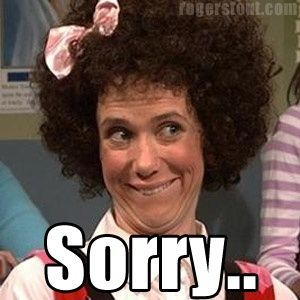